History of C Language
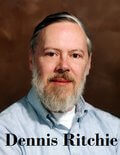
History of C language is interesting to know. Here we are going to discuss a brief history of the c language.
C programming language was developed in 1972 by Dennis Ritchie at bell laboratories of AT&T (American Telephone & Telegraph), located in the U.S.A.
Dennis Ritchie is known as the founder of the c language.
C is a general-purpose programming language that is extremely popular, simple and flexible. It is machine-independent, structured programming language which is used extensively in various applications.
what is c language?????
C was the basics language to write everything from operating systems (Windows and many others) to complex programs like the Oracle database, Git, Python interpreter and more.
It is said that 'C' is a god's programming language. One can say, C is a base for the programming. If you know 'C,' you can easily grasp the knowledge of the other programming languages that uses the concept of 'C'
It is essential to have a background in computer memory mechanisms because it is an important aspect when dealing with the C programming language.
ANSI C provides three types of data types:
- Primary(Built-in) Data Types: void, int, char, double and float.
- Derived Data Types: Array, References, and Pointers.
- User Defined Data Types: Structure, Union, and Enumeration.
- what is constant????
Constants are like a variable, except that their value never changes during execution once defined. ... Constants in C are the fixed values that are used in a program, and its value remains the same during the entire execution of the program. Constants are also called literals. Constants can be any of the data types.
what are variables??
A variable is nothing but a name given to a storage area that our programs can manipulate. Each variable in C has a specific type, which determines the size and layout of the variable's memory; the range of values that can be stored within that memory; and the set of operations that can be applied to the variable.
TYPES OF OPERATORS IN C
ARITHMETIC OPERATORS
ASSIGNMENT OPERATORS
INCREMENT AND DECREMENT
RELATIONAL OPERATORS
LOGICAL OPERATORS
An operator is a symbol that operates on a value or a variable. For example: + is an operator to perform addition.
C has a wide range of operators to perform various operations.
C Arithmetic Operators
An arithmetic operator performs mathematical operations such as addition, subtraction, multiplication, division etc on numerical values (constants and variables).
C Increment and Decrement Operators
C programming has two operators increment
++
and decrement --
to change the value of an operand (constant or variable) by 1.
Increment
++
increases the value by 1 whereas decrement --
decreases the value by 1. These two operators are unary operators, meaning they only operate on a single operand.C Assignment Operators
An assignment operator is used for assigning a value to a variable. The most common assignment operator is
=
+=
-=
*=
/= ETC.
Example 3: Assignment Operators
// Working of assignment operators
#include <stdio.h>
int main()
{
int a = 5, c;
c = a; // c is 5
printf("c = %d\n", c);
c += a; // c is 10
printf("c = %d\n", c);
c -= a; // c is 5
printf("c = %d\n", c);
c *= a; // c is 25
printf("c = %d\n", c);
c /= a; // c is 5
printf("c = %d\n", c);
c %= a; // c = 0
printf("c = %d\n", c);
return 0;
}
Output
c = 5
c = 10
c = 5
c = 25
c = 5
c = 0
// Working of assignment operators
#include <stdio.h>
int main()
{
int a = 5, c;
c = a; // c is 5
printf("c = %d\n", c);
c += a; // c is 10
printf("c = %d\n", c);
c -= a; // c is 5
printf("c = %d\n", c);
c *= a; // c is 25
printf("c = %d\n", c);
c /= a; // c is 5
printf("c = %d\n", c);
c %= a; // c = 0
printf("c = %d\n", c);
return 0;
}
C Relational Operators
A relational operator checks the relationship between two operands. If the relation is true, it returns 1; if the relation is false, it returns value 0.
Relational operators are used in decision making and loops.
Operator | Meaning of Operator | Example |
---|---|---|
== | Equal to | 5 == 3 is evaluated to 0 |
> | Greater than | 5 > 3 is evaluated to 1 |
< | Less than | 5 < 3 is evaluated to 0 |
!= | Not equal to | 5 != 3 is evaluated to 1 |
>= | Greater than or equal to | 5 >= 3 is evaluated to 1 |
<= | Less than or equal to | 5 <= 3 is evaluated to 0 |
Example 4: Relational Operators
// Working of relational operators
#include <stdio.h>
int main()
{
int a = 5, b = 5, c = 10;
printf("%d == %d is %d \n", a, b, a == b);
printf("%d == %d is %d \n", a, c, a == c);
printf("%d > %d is %d \n", a, b, a > b);
printf("%d > %d is %d \n", a, c, a > c);
printf("%d < %d is %d \n", a, b, a < b);
printf("%d < %d is %d \n", a, c, a < c);
printf("%d != %d is %d \n", a, b, a != b);
printf("%d != %d is %d \n", a, c, a != c);
printf("%d >= %d is %d \n", a, b, a >= b);
printf("%d >= %d is %d \n", a, c, a >= c);
printf("%d <= %d is %d \n", a, b, a <= b);
printf("%d <= %d is %d \n", a, c, a <= c);
return 0;
}
Output
5 == 5 is 1
5 == 10 is 0
5 > 5 is 0
5 > 10 is 0
5 < 5 is 0
5 < 10 is 1
5 != 5 is 0
5 != 10 is 1
5 >= 5 is 1
5 >= 10 is 0
5 <= 5 is 1
5 <= 10 is 1
// Working of relational operators
#include <stdio.h>
int main()
{
int a = 5, b = 5, c = 10;
printf("%d == %d is %d \n", a, b, a == b);
printf("%d == %d is %d \n", a, c, a == c);
printf("%d > %d is %d \n", a, b, a > b);
printf("%d > %d is %d \n", a, c, a > c);
printf("%d < %d is %d \n", a, b, a < b);
printf("%d < %d is %d \n", a, c, a < c);
printf("%d != %d is %d \n", a, b, a != b);
printf("%d != %d is %d \n", a, c, a != c);
printf("%d >= %d is %d \n", a, b, a >= b);
printf("%d >= %d is %d \n", a, c, a >= c);
printf("%d <= %d is %d \n", a, b, a <= b);
printf("%d <= %d is %d \n", a, c, a <= c);
return 0;
}
C Logical Operators
An expression containing logical operator returns either 0 or 1 depending upon whether expression results true or false. Logical operators are commonly used in decision making in C programming.
Operator | Meaning | Example |
---|---|---|
&& | Logical AND. True only if all operands are true | If c = 5 and d = 2 then, expression ((c==5) && (d>5)) equals to 0. |
|| | Logical OR. True only if either one operand is true | If c = 5 and d = 2 then, expression ((c==5) || (d>5)) equals to 1. |
! | Logical NOT. True only if the operand is 0 | If c = 5 then, expression !(c==5) equals to 0. |
Example 5: Logical Operators
// Working of logical operators
#include <stdio.h>
int main()
{
int a = 5, b = 5, c = 10, result;
result = (a == b) && (c > b);
printf("(a == b) && (c > b) is %d \n", result);
result = (a == b) && (c < b);
printf("(a == b) && (c < b) is %d \n", result);
result = (a == b) || (c < b);
printf("(a == b) || (c < b) is %d \n", result);
result = (a != b) || (c < b);
printf("(a != b) || (c < b) is %d \n", result);
result = !(a != b);
printf("!(a == b) is %d \n", result);
result = !(a == b);
printf("!(a == b) is %d \n", result);
return 0;
}
Output
(a == b) && (c > b) is 1
(a == b) && (c < b) is 0
(a == b) || (c < b) is 1
(a != b) || (c < b) is 0
!(a != b) is 1
!(a == b) is 0
Explanation of logical operator program
(a == b) && (c > 5)
evaluates to 1 because both operands (a == b)
and (c > b)
is 1 (true).
(a == b) && (c < b)
evaluates to 0 because operand (c < b)
is 0 (false).
(a == b) || (c < b)
evaluates to 1 because (a = b)
is 1 (true).
(a != b) || (c < b)
evaluates to 0 because both operand (a != b)
and (c < b)
are 0 (false).
!(a != b)
evaluates to 1 because operand (a != b)
is 0 (false). Hence, !(a != b) is 1 (true).
!(a == b)
evaluates to 0 because (a == b)
is 1 (true). Hence, !(a == b)
is 0 (false).
// Working of logical operators
#include <stdio.h>
int main()
{
int a = 5, b = 5, c = 10, result;
result = (a == b) && (c > b);
printf("(a == b) && (c > b) is %d \n", result);
result = (a == b) && (c < b);
printf("(a == b) && (c < b) is %d \n", result);
result = (a == b) || (c < b);
printf("(a == b) || (c < b) is %d \n", result);
result = (a != b) || (c < b);
printf("(a != b) || (c < b) is %d \n", result);
result = !(a != b);
printf("!(a == b) is %d \n", result);
result = !(a == b);
printf("!(a == b) is %d \n", result);
return 0;
}
(a == b) && (c > 5)
evaluates to 1 because both operands (a == b)
and (c > b)
is 1 (true).(a == b) && (c < b)
evaluates to 0 because operand (c < b)
is 0 (false).(a == b) || (c < b)
evaluates to 1 because (a = b)
is 1 (true).(a != b) || (c < b)
evaluates to 0 because both operand (a != b)
and (c < b)
are 0 (false).!(a != b)
evaluates to 1 because operand (a != b)
is 0 (false). Hence, !(a != b) is 1 (true).!(a == b)
evaluates to 0 because (a == b)
is 1 (true). Hence, !(a == b)
is 0 (false).C Bitwise Operators
During computation, mathematical operations like: addition, subtraction, multiplication, division, etc are converted to bit-level which makes processing faster and saves power.
Bitwise operators are used in C programming to perform bit-level operations.
Operators | Meaning of operators |
---|---|
& | Bitwise AND |
| | Bitwise OR |
^ | Bitwise exclusive OR |
~ | Bitwise complement |
<< | Shift left |
>> | Shift right |
Visit bitwise operator in C to learn more.
Other Operators
Comma Operator
For loop
A for loop is a more efficient loop structure in 'C' programming. The general structure of for loop is as follows:
for (initial value; condition; incrementation or decrementation ) { statements; }
- The initial value of the for loop is performed only once.
- The condition is a Boolean expression that tests and compares the counter to a fixed value after each iteration, stopping the for loop when false is returned.
- The incrementation/decrementation increases (or decreases) the counter by a set value.
Following program illustrates the use of a simple for loop:
#include<stdio.h> int main() { int number; for(number=1;number<=10;number++) //for loop to print 1-10 numbers { printf("%d\n",number); //to print the number } return 0; }
Output:
While Loop
A while loop is the most straightforward looping structure. The basic format of while loop is as follows:
while (condition) { statements; }
It is an entry-controlled loop. In while loop, a condition is evaluated before processing a body of the loop. If a condition is true then and only then the body of a loop is executed. After the body of a loop is executed then control again goes back at the beginning, and the condition is checked if it is true, the same process is executed until the condition becomes false. Once the condition becomes false, the control goes out of the loop.
After exiting the loop, the control goes to the statements which are immediately after the loop. The body of a loop can contain more than one statement. If it contains only one statement, then the curly braces are not compulsory. It is a good practice though to use the curly braces even we have a single statement in the body.
In while loop, if the condition is not true, then the body of a loop will not be executed, not even once. It is different in do while loop which we will see shortly.
Following program illustrates a while loop:
#include<stdio.h> #include<conio.h> int main() { int num=1; //initializing the variable while(num<=10) //while loop with condition { printf("%d\n",num); num++; //incrementing operation } return 0; }
Output:
Do-While loop
A do-while loop is similar to the while loop except that the condition is always executed after the body of a loop. It is also called an exit-controlled loop.
The basic format of while loop is as follows:
do { statements } while (expression);
As we saw in a while loop, the body is executed if and only if the condition is true. In some cases, we have to execute a body of the loop at least once even if the condition is false. This type of operation can be achieved by using a do-while loop.
In the do-while loop, the body of a loop is always executed at least once. After the body is executed, then it checks the condition. If the condition is true, then it will again execute the body of a loop otherwise control is transferred out of the loop.
Similar to the while loop, once the control goes out of the loop the statements which are immediately after the loop is executed.
The critical difference between the while and do-while loop is that in while loop the while is written at the beginning. In do-while loop, the while condition is written at the end and terminates with a semi-colon (;)
The following program illustrates the working of a do-while loop:
We are going to print a table of number 2 using do while loop.
#include<stdio.h> #include<conio.h> int main() { int num=1; //initializing the variable do //do-while loop { printf("%d\n",2*num); num++; //incrementing operation }while(num<=10); return 0; }
Output:
WHAT IS POINTER??
Pointers in C language is a variable that stores/points the address of another variable. A Pointer in C is used to allocate memory dynamically i.e. at run time. The pointer variable might be belonging to any of the data type such as int, float, char, double, short etc
WHAT IS ARRAY??
An array in C or C++ is a collection of items stored at contiguous memory locations and elements can be accessed randomly using indices of an array. They are used to store similar type of elements as in the data type must be the same for all elements.
There are 2 types of C arrays. They are,
- One dimensional array.
- Multi dimensional array. Two dimensional array. Three dimensional array. four dimensional array etc
C switch Statement
In this tutorial, you will learn to create the switch statement in C programming with the help of an example.
The switch statement allows us to execute one code block among many alternatives.
You can do the same thing with the
if...else..if
ladder. However, the syntax of the switch
statement is much easier to read and write.Syntax of switch...case
switch (expression)
​{
case constant1:
// statements
break;
case constant2:
// statements
break;
.
.
.
default:
// default statements
}
How does the switch statement work?
The expression is evaluated once and compared with the values of each case label.
- If there is a match, the corresponding statements after the matching label are executed. For example, if the value of the expression is equal to constant2, statements after
case constant2:
are executed untilbreak
is encountered. - If there is no match, the default statements are executed.
If we do not use
break
, all statements after the matching label are executed.
By the way, the
default
clause inside the switch
statement is optional.
BREAK STATEMENT
C break statement. The break is a keyword in C which is used to bring the program control out of the loop. The break statement is used inside loops or switch statement. The break statement breaks the loop one by one, i.e., in the case of nested loops, it breaks the inner loop first and then proceeds to outer loops.
CONTINUE STATEMENT IN C
C Functions
In c, we can divide a large program into the basic building blocks known as function. The function contains the set of programming statements enclosed by {}. A function can be called multiple times to provide reusability and modularity to the C program. In other words, we can say that the collection of functions creates a program. The function is also known as procedure or subroutine in other programming languages.
Advantage of functions in C
There are the following advantages of C functions.
- By using functions, we can avoid rewriting same logic/code again and again in a program.
- We can call C functions any number of times in a program and from any place in a program.
- We can track a large C program easily when it is divided into multiple functions.
- Reusability is the main achievement of C functions.
- However, Function calling is always a overhead in a C program.
Function Aspects
There are three aspects of a C function.
- Function declaration A function must be declared globally in a c program to tell the compiler about the function name, function parameters, and return type.
- Function call Function can be called from anywhere in the program. The parameter list must not differ in function calling and function declaration. We must pass the same number of functions as it is declared in the function declaration.
- Function definition It contains the actual statements which are to be executed. It is the most important aspect to which the control comes when the function is called. Here, we must notice that only one value can be returned from the function.
SN | C function aspects | Syntax |
---|---|---|
1 | Function declaration | return_type function_name (argument list); |
2 | Function call | function_name (argument_list) |
3 | Function definition | return_type function_name (argument list) {function body;} |
The syntax of creating function in c language is given below:
Types of Functions
There are two types of functions in C programming:
- Library Functions: are the functions which are declared in the C header files such as scanf(), printf(), gets(), puts(), ceil(), floor() etc.
- User-defined functions: are the functions which are created by the C programmer, so that he/she can use it many times. It reduces the complexity of a big program and optimizes the code.
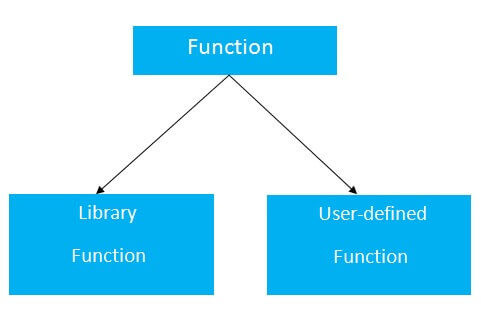
Return Value
A C function may or may not return a value from the function. If you don't have to return any value from the function, use void for the return type.
Let's see a simple example of C function that doesn't return any value from the function.
Example without return value:
If you want to return any value from the function, you need to use any data type such as int, long, char, etc. The return type depends on the value to be returned from the function.
Let's see a simple example of C function that returns int value from the function.
Example with return value:
In the above example, we have to return 10 as a value, so the return type is int. If you want to return floating-point value (e.g., 10.2, 3.1, 54.5, etc), you need to use float as the return type of the method.
Now, you need to call the function, to get the value of the function.
Different aspects of function calling
A function may or may not accept any argument. It may or may not return any value. Based on these facts, There are four different aspects of function calls.
- function without arguments and without return value
- function without arguments and with return value
- function with arguments and without return value
- function with arguments and with return value
Example for Function without argument and return value
Example 1
Output
Hello Javatpoint
Example 2
Output
Going to calculate the sum of two numbers: Enter two numbers 10 24 The sum is 34
Example for Function without argument and with return value
Example 1
Output
Going to calculate the sum of two numbers: Enter two numbers 10 24 The sum is 34
Example 2: program to calculate the area of the square
Output
Going to calculate the area of the square Enter the length of the side in meters: 10 The area of the square: 100.000000
Example for Function with argument and without return value
Example 1
Output
Going to calculate the sum of two numbers: Enter two numbers 10 24 The sum is 34
Example 2: program to calculate the average of five numbers.
Output
Going to calculate the average of five numbers: Enter five numbers:10 20 30 40 50 The average of given five numbers : 30.000000
Example for Function with argument and with return value
Example 1
Output
Going to calculate the sum of two numbers: Enter two numbers:10 20 The sum is : 30
Example 2: Program to check whether a number is even or odd
Output
Going to check whether a number is even or odd Enter the number: 100 The number is even
C Library Functions
Library functions are the inbuilt function in C that are grouped and placed at a common place called the library. Such functions are used to perform some specific operations. For example, printf is a library function used to print on the console. The library functions are created by the designers of compilers. All C standard library functions are defined inside the different header files saved with the extension .h. We need to include these header files in our program to make use of the library functions defined in such header files. For example, To use the library functions such as printf/scanf we need to include stdio.h in our program which is a header file that contains all the library functions regarding standard input/output.
The list of mostly used header files is given in the following table.
SN | Header file | Description |
---|---|---|
1 | stdio.h | This is a standard input/output header file. It contains all the library functions regarding standard input/output. |
2 | conio.h | This is a console input/output header file. |
3 | string.h | It contains all string related library functions like gets(), puts(),etc. |
4 | stdlib.h | This header file contains all the general library functions like malloc(), calloc(), exit(), etc. |
5 | math.h | This header file contains all the math operations related functions like sqrt(), pow(), etc. |
6 | time.h | This header file contains all the time-related functions. |
7 | ctype.h | This header file contains all character handling functions. |
8 | stdarg.h | Variable argument functions are defined in this header file. |
9 | signal.h | All the signal handling functions are defined in this header file. |
10 | setjmp.h | This file contains all the jump functions. |
11 | locale.h | This file contains locale functions. |
12 | errno.h | This file contains error handling functions. |
13 | assert.h | This file contains diagnostics functions. |